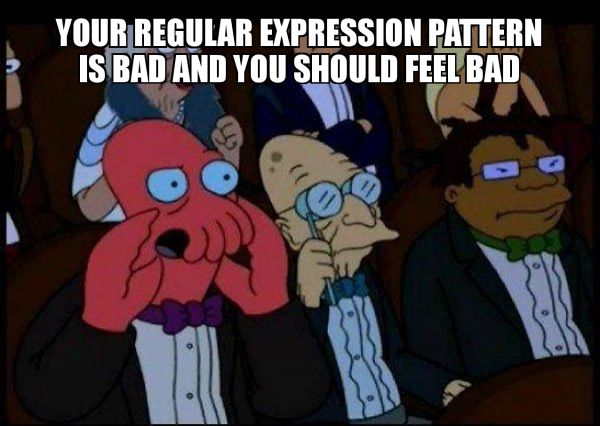
Regular expwhat??
Regular expressions are patterns used to match character combinations in strings. In JavaScript, regular expressions are also objects. These patterns are used with the exec() and test() methods of RegExp and with match(), matchAll(), replace(), replaceAll(), search() and split() methods for Strings.
Creating a Regular Expression:
You construct a regular expression in one of two ways:
- Regular expression literals provide compilation of the regular expression when the script is loaded. If the regular expression remains constant, using this can improve performance.Using a regular expression literal, which consists of a pattern enclosed between slashes, as follows:Copy to Clipboard
const re = /ab+c/;
- Using the constructor function provides runtime compilation of the regular expression. Use the constructor function when you know the regular expression pattern will be changing, or you don't know the pattern and are getting it from another source, such as user input.Or calling the constructor function of the
RegExp
object, as follows:Copy to Clipboard
// syntax:
const regexp = new RegExp("pattern", "flags");
// example:
const re = new RegExp("ab+c", "i");
// or
const re = new RegExp(/ab+c/i);
* Using the constructor function provides runtime compilation of the regular expression. Use the constructor function when you know the regular expression pattern will be changing, or you don't know the pattern and are getting it from another source, such as user input.
String methods
search()
Uses an expression to search for a match, and returns the position of the match. Can use a "string" or a regular expression.
let text = "Visit W3Schools!";
let n = text.search("W3Schools"); // 6
replace()
Returns a modified string where the pattern is replaced. Can use a "string" or a regular expression.
const example = 'I love you'
const result = example.replace('love', 'like') // I like you
The second parameter accepts symbols that modify the behaviour:
$&
inserts the whole match$`
inserts the part of the string before the match$'
inserts the part of the string after the match$n
if "n" is a 1-2 digit number, then it inserts the contents of n-th parentheses (capturing groups)$<name>
inserts the contents of the parentheses with the givenname
(capturing groups)$$
inserts character$
"I love HTML".replace(/HTML/, "$& and JavaScript")
split()
takes a pattern and divides a String into an ordered list of substrings.
const example = 'what am I doing here?'
const words = example.split(' ') // ["what", "am", "doing", "here?"]
match()
Retrieves the result of matching a string against a regular expression.
const paragraph = 'The quick brown fox jumps over the lazy dog. It barked.';
const regex = /[A-Z]/g;
const found = paragraph.match(regex); // Expected output: Array ["T", "I"]
Regular expression methods
test()
Searches a string for a pattern, and returns true or false, depending on the result.
const pattern = /e/;
pattern.test("The best things in life are free!"); // true
exec()
Searches a string for a specified pattern, and returns the found text as an object.
const ex = /u ag/.exec('who are you again???')
console.log(ex) // ["u ag"]
Don't be shy, leave us a comment